I'm trying to store the data in ScaleOut and I am able to save the String value. And I am able to validate the stored data in the ScaleOut through ScaleOut Object Browser.
But when I am trying to store the class object in ScaleOut, code is successfully executing. But when I'm trying to validate the stored data in the scaleout through ScaleOut Object Browser, I'm getting the object deserialization error.
Error:
System.Reflection.TargetInvocationException: Exception has been thrown by the target of an invocation. ---> System.Runtime.Serialization.SerializationException: There was an error deserializing the object . The data at the root level is invalid. Line 1, position 1. ---> System.Xml.XmlException: The data at the root level is invalid. Line 1, position 1.
at System.Xml.XmlExceptionHelper.ThrowXmlException(XmlDictionaryReader reader, String res, String arg1, String arg2, String arg3)
at System.Xml.XmlUTF8TextReader.Read()
at System.Xml.XmlBaseReader.IsStartElement()
at System.Runtime.Serialization.NetDataContractSerializer.InternalReadObject(XmlReaderDelegator xmlReader, Boolean verifyObjectName)
at System.Runtime.Serialization.XmlObjectSerializer.ReadObjectHandleExceptions(XmlReaderDelegator reader, Boolean verifyObjectName, DataContractResolver dataContractResolver)
--- End of inner exception stack trace ---
at System.Runtime.Serialization.XmlObjectSerializer.ReadObjectHandleExceptions(XmlReaderDelegator reader, Boolean verifyObjectName, DataContractResolver dataContractResolver)
at System.Runtime.Serialization.XmlObjectSerializer.ReadObject(XmlDictionaryReader reader)
--- End of inner exception stack trace ---
at System.RuntimeMethodHandle.InvokeMethod(Object target, Object[] arguments, Signature sig, Boolean constructor)
at System.Reflection.RuntimeMethodInfo.UnsafeInvokeInternal(Object obj, Object[] parameters, Object[] arguments)
at System.Reflection.RuntimeMethodInfo.Invoke(Object obj, BindingFlags invokeAttr, Binder binder, Object[] parameters, CultureInfo culture)
at DotNetBrowser.CustomDeserializationConfig.Deserialize(Stream stream)
at DotNetBrowser.UserControls.ObjectPane.DeserializeObject(MemoryStream stream)
But when I am trying to store the class object in ScaleOut, code is successfully executing. But when I'm trying to validate the stored data in the scaleout through ScaleOut Object Browser, I'm getting the object deserialization error.
Error:
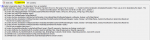
System.Reflection.TargetInvocationException: Exception has been thrown by the target of an invocation. ---> System.Runtime.Serialization.SerializationException: There was an error deserializing the object . The data at the root level is invalid. Line 1, position 1. ---> System.Xml.XmlException: The data at the root level is invalid. Line 1, position 1.
at System.Xml.XmlExceptionHelper.ThrowXmlException(XmlDictionaryReader reader, String res, String arg1, String arg2, String arg3)
at System.Xml.XmlUTF8TextReader.Read()
at System.Xml.XmlBaseReader.IsStartElement()
at System.Runtime.Serialization.NetDataContractSerializer.InternalReadObject(XmlReaderDelegator xmlReader, Boolean verifyObjectName)
at System.Runtime.Serialization.XmlObjectSerializer.ReadObjectHandleExceptions(XmlReaderDelegator reader, Boolean verifyObjectName, DataContractResolver dataContractResolver)
--- End of inner exception stack trace ---
at System.Runtime.Serialization.XmlObjectSerializer.ReadObjectHandleExceptions(XmlReaderDelegator reader, Boolean verifyObjectName, DataContractResolver dataContractResolver)
at System.Runtime.Serialization.XmlObjectSerializer.ReadObject(XmlDictionaryReader reader)
--- End of inner exception stack trace ---
at System.RuntimeMethodHandle.InvokeMethod(Object target, Object[] arguments, Signature sig, Boolean constructor)
at System.Reflection.RuntimeMethodInfo.UnsafeInvokeInternal(Object obj, Object[] parameters, Object[] arguments)
at System.Reflection.RuntimeMethodInfo.Invoke(Object obj, BindingFlags invokeAttr, Binder binder, Object[] parameters, CultureInfo culture)
at DotNetBrowser.CustomDeserializationConfig.Deserialize(Stream stream)
at DotNetBrowser.UserControls.ObjectPane.DeserializeObject(MemoryStream stream)
Last edited: